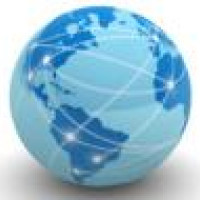
Un buon sito si costruisce prima di tutto creandolo con funzionalità multilingua. Ovvero, sulla base dell'IP dell'utente, cercare di mandare in output il sito nel suo linguaggio senza troppi fronzoli tecnici.
Questo si traduce, nella pratica, nella creazione di un sito con una buona classe di traduzione delle stringhe on-the-fly, un pò come fa wordpress. Per esempio, wordpress fa uso dei file di traduzione *.po e *.mo.. Si definisce una stringa, con la sua "chiave primaria" nel linguaggio che si vuole, ad esempio:
<div id="test"><?php echo __ ( 'This is the main key for the string "this is the main key for the string"' ); ?></div>
A questo punto, aiutandovi con le funzioni di output buffering, e sulla base della geolocation dell'IP dell'utente che sta visualizzando il sito, potete mandare in output la traduzione corretta della stringa This is the main key for the string "this is the main key for the string"
In internet esistono svariate classi che permettono la geolocation. A me personalmente non piace fare le cose semplici, e mi piace avere la situazione sotto controllo. Quindi, mi sono documentato, e ho trovato un sito che permette il download di un file di mapping tra indirizzi IP e stati.
Avendo in mano un file del genere, è possibile creare un DB che abbia le referenziazioni tra IP e location, e così possiamo dare sfogo alla nostra creatività in termini di output da mandare all'utente ^^
Prima di tutto, prepariamo i DB che contengono i dati del DB IP-to-country
CREATE TABLE IF NOT EXISTS `ip_to_country` (
`id` int(11) NOT NULL auto_increment,
`ip_start_integer` int(11) NOT NULL,
`ip_end_integer` int(11) NOT NULL,
`country_id` int(11) NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `ip_start_integer_index` (`ip_start_integer`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
CREATE TABLE IF NOT EXISTS `country_list` (
`id` int(11) NOT NULL auto_increment,
`country_code` varchar(10) character set utf8 collate utf8_bin NOT NULL,
`country_name_en` varchar(255) character set utf8 collate utf8_bin NOT NULL,
`country_name_it` varchar(255) character set utf8 collate utf8_bin NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `country-name` (`country_name_en`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Adesso, passiamo alla parte divertente, cioè al parsing del file di mapping IP-country e al relativo salvataggio dei dati nelle nostre 2 tabelle mysql
<?php
/*
http://www.ipinfodb.com/
API KEY: bisogna registrarsi per averla
http://lite.ip2location.com/?file=IP2LOCATION-LITE-DB1.CSV.ZIP&key=
*/
// CONNESSIONE AL DB - DA INSERIRE QUI
$stream_ZIPFILE = __CURL_getURL( 'http://lite.ip2location.com/?file=IP2LOCATION-LITE-DB1.CSV.ZIP&key=la-chiave-api-del-sito-ipinfodb.com', 'http://www.ipinfodb.com/download.php?file=IP2LOCATION-LITE-DB1.CSV.ZIP' );
file_put_contents ( 'tempzipfile', $stream_ZIPFILE );
$stream_ZIPFILE = ''; // releasing memory for this variable
$zip = zip_open ( 'tempzipfile' );
unlink ( 'tempzipfile' );
do {
$entry = zip_read($zip);
} while ($entry && zip_entry_name($entry) != "IP2LOCATION-LITE-DB1.CSV");
zip_entry_open($zip, $entry, "r");
$entry_content = zip_entry_read($entry, zip_entry_filesize($entry));
$entry_content = str_replace ( chr(13).chr(10), chr(10), $entry_content );
$linee = explode ( chr(10), $entry_content );
$entry_content = ''; // releasing memory for entry_content ( it's a long string )
// flushing ip_to_country DB
$sql = "TRUNCATE TABLE ip_to_country";
mysql_query($sql);
// parsing Text DB Lines
foreach ( $linee as $linea ) {
list ( $start_ip_integer, $end_ip_integer, $country_code, $country_name ) = explode ( ',', str_replace ( '"', '' , $linea ) );
// inserting country into country DB (if needed)
$sql = "SELECT id FROM country_list WHERE country_name_en='" . $country_name . "'";
$rs = mysql_query ( $sql );
if ( $rs && mysql_num_rows ( $rs ) == 1 ) $idCountry = mysql_result ( $rs, 0 );
else {
// translating country in Italian
$stream_TRANSLATE = __CURL_getURL( 'https://www.googleapis.com/language/translate/v2?key=la-tua-api-key-google.translate&q=' . urlencode ( $country_name ) . '&source=en&target=it', 'http://www.google.it/' );
$stream_TRANSLATE_array = json_decode ( $stream_TRANSLATE );
$countryname_IT = strtoupper ( $stream_TRANSLATE_array -> data -> translations[0] -> translatedText );
$sql = "INSERT INTO country_list ( country_code, country_name_en, country_name_it ) VALUES ( '$country_code', '$country_name', '$countryname_IT' )";
mysql_query ( $sql );
$idCountry = mysql_insert_id();
}
$sql = "INSERT INTO ip_to_country ( ip_start_integer, ip_end_integer, country_id ) VALUES ( '$start_ip_integer', '$end_ip_integer', '$idCountry' )";
mysql_query ( $sql );
}
function __CURL_getURL($url, $referer) {
$header[0] = "Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8";
$header[] = "Cache-Control: max-age=0";
$header[] = "Connection: keep-alive";
$header[] = "Keep-Alive: 300";
$header[] = "Accept-Charset: ISO-8859-1,utf-8;q=0.7,*;q=0.7";
$header[] = "Accept-Language: it-it,it;q=0.8,en-us;q=0.5,en;q=0.3";
$header[] = "Pragma: ";
$header[] = "Referer: " . $referer;
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $header);
curl_setopt($ch, CURLOPT_USERAGENT, "Mozilla/5.0 (Windows; U; Windows NT 5.1; it; rv:1.9.1.3) Gecko/20090824 Firefox/3.5.3");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_VERBOSE, true);
curl_setopt($ch, CURLOPT_COOKIEJAR, '/tmp/cookies.txt');
curl_setopt($ch, CURLOPT_COOKIEFILE, '/tmp/cookies.txt');
$result = curl_exec($ch);
curl_close($ch);
return $result;
}
?>
Per avviare questo codice, avrete bisogno di 2 api key:
- Una api key del servizio http://www.ipinfodb.com/ - rimpiazza la stringa "la-chiave-api-del-sito-ipinfodb.com" all'interno del sorgente qui sopra con la tua api key
- Una api key del servizio Google Translate API - rimpiazza la stringa "la-tua-api-key-google.translate" all'interno del sorgente qui sopra con la tua api key
Una volta fatto tutto, potete schedulare questo script per l'esecuzione automatica con un cronjob. Anche una volta alla settimana va bene, non è necessario farlo partire ogni ora ^^
Ora, ti starai chiedendo: e come faccio a stabilire la geolocation dell'utente con questi due database? Bene, ecco le poche linee di codice per sfruttare le tabelle.
// ottenere la "country_id" dal database delle country per un particolare IP
$ip = $_SERVER['REMOTE_ADDR']; // o qualsiasi ip nel formato 123.123.123.123 ottenuto con qualsiasi metodo
$sql = "SELECT country_id FROM ip_to_country WHERE ip_start_integer <= " . ip2long ( $ip ) . " ORDER BY ip_start_integer DESC LIMIT 1";
$rs = mysql_query ( $sql );
if ( $rs ) $country_id = mysql_result ( $rs, 0 );
Con la country_id per un particolare IP, sarà vostro compito stabilire quale lingua mandare in output all'utente.
Ovviamente, questo database può avere tantissimi risvolti pratici. Un altro che mi viene in mente è la geolocalizzazione di server proxy, o la geolocalizzazione degli IP di una applicazione di tracciamento degli utenti.
Insomma, con le tabelle IP-to-country avrete a disposizione tantissime possibilità di sviluppo e soprattutto, avendo in mano i dati nel vostro DB, potrete generare i volumi di traffico che desiderate per quanto riguarda le geolocation calcolate per ora. Infatti, alcuni servizi online di geolocalizzazione, vi impediscono di fare più di un tot di query all'ora.
Ultima modifica: Lunedì 9 Mag 2011, alle 22:22